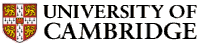 |
Department of Engineering |
 |
 |
Next: Reading Directories
Up: Examples
Previous: Using pointers instead of
Contents
The program reads from stdin an ASCII file containing values
of a variable y for integral values of x running from 0
to n-1 where n is the number of values in the file.
There may be several values on each line. The program outputs
the x, y pairs, one pair per line, the y values
scaled and translated by factors built into the main routine..
#include <stdio.h>
#include <stdlib.h>
int answer;
float offset;
float scale;
char buf[BUFSIZ];
int xcoord = 0;
char *cptr;
int transform(int a)
{
return a * scale + offset + 0.5;
}
char* eat_space(char *cptr){
/* This while loop skips to the nonspace after spaces.
If this is the end of the line, return NULL
`While a space, keep going'
*/
while (*cptr ==' '){
if (*cptr == '\0')
return NULL;
else
cptr++;
}
return cptr;
}
char * next_next_num(char *cptr){
/* This while loop skips to the 1st space after a number.
If this is the end of the line, return NULL
`While NOT a space, keep going'
*/
while (*cptr !=' '){
if (*cptr == '\0')
return NULL;
else
cptr++;
}
/* Now move to the start of the next number */
return eat_space(cptr);
}
int main(int argc, char *argv[])
{
offset = 2.3;
scale = 7.5;
while(1){
/* if we haven't reached the end of the file ...*/
if(fgets(buf, BUFSIZ,stdin)!= NULL){
/* initialise cptr to point to the first number ...*/
cptr = eat_space(buf);
do{
/* convert the representation of the num into an int */
sscanf(cptr,"%d", &num);
/* print x and y to stdout */
printf("%d %d\n",xcoord, tranform(num));
/* skip to the start of the next number on the line */
cptr=next_next_num(cptr);
xcoord++;
}while ( cptr!=NULL);
}
else{
exit(0);
}
}
}
Tim Love
2010-04-27