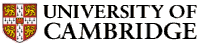 |
Department of Engineering |
 |
 |
Java Notes
Introduction
Java(tm) is an Object Oriented platform independent programming
language from Sun. It was designed to be a "simple, object-orientated,
distributed, interpreted, robust, secure, architecture neutral, portable,
high-performance, multithreaded, and dynamic language". At a low level
the source looks rather like C++. Unlike C++ however, it has standard
graphical and internet routines. Also it's more purely object-orientated -
it's a new language that didn't have to try to be compatible with C.
Jargon
- JavaBeans - a reusable software component architecture, like VBX
(Visual Basic Extensions) or OCX (OLE Controls). JavaBeans components -
beans - are separate code modules, written at the source level, that an
application developer can use to combine into larger applications. You can
make any Java class into a bean just by changing the class to adhere to the
JavaBeans specification.
- Java Server Pages - it embeds Java code in HTML.
- Applets - java designed to be run in a WWW browser.
- Servlets - Java's replacement for CGI scripts (embeds HTML in java).
- Swing - a set of libraries for making GUIs
- Packages - namespaces for classes (sort of)
Language basics
keywords:
abstract default if private throw
boolean do implements protected throws
break double import public transient
byte else instanceof return try
case extends int short void
catch final interface static volatile
char finally long super while
class float native switch
const for new synchronized
continue goto package this
All primitive values belong to one of eight primitive types: int, float, boolean, char, byte, short, long, and double.
Program Structure
As you may already have guessed from the keyword list,
Java has many features in common with C++. Every Java program consists of a
collection of classes - nothing else. A class is a template for creating a
particular form of object. Each object
created by the template contains the same members, each of which is either a field or a method. A field is a "container" that holds a value. A
method is an operation on the fields of the object and any values that are passed as arguments to the method. The objects created by a particular
class template are called the instances or objects of that class. Each instance contains the members specified in the class template.
Every complete Java program must contain a root class where execution can begin. A root class must contain a main method defined with the header
public static void main(String[] args)
A simple example
Put the following into a file called Euro.java.
class Euro {
public static void main(String[] args) {
System.out.println("99 euros = " + 99. *(61./100.) + " pounds");
}
}
This has a root class which prints out 2 strings and the result of a
calculation by calling the println method.
With C++, the source code is compiled to produce a file in the
native language of the CPU. With interpreted languages like BASIC
the source is converted at run-time (almost line-by-line) by an
interpreter. Java is both compiled and interpreted.
To execute this it first needs to be compiled into a byte-code
file. The Java compiler, javac, takes your
source file and translates its text into the native language
of the Java Virtual Machine.
javac euro.java
This produces a file called Euro.class which can be fed to the
java interpreter by typing
java Euro.class
Applets and Inheritance
As in C++, classes can be derived from other classes. A simple
example of this is applet creation.
An applet is a piece of java that can be run inside a java-enabled
browser. To give a class access to the functions that an applet
needs, it's derived from an Applet class.
Put the following into a file called EuroApplet.java
somewhere on the WWW.
import java.applet.Applet;
import java.awt.Graphics;
public class EuroApplet extends Applet {
public void paint(Graphics g) {
g.drawString("Hello world!", 50, 25);
}
}
Then create a WWW page with the following code on it
<applet codebase="http://the.folder.where.your.EuroApplet.is"
code="EuroApplet.class" width=400 height=75 align=center >
<param name="text" value="This is the Applet Viewer.">
<blockquote>
<hr>
If you were using a Java-enabled browser,
you would see dancing text instead of this paragraph.
<hr>
</blockquote>
</applet>
When you view the page you should see the applet's output too.
Java Utilities
The suite of java utilities includes
- Java Compiler (javac)
- Java Interpreter (java)
Further Information